静态绑定
通过添加数组.xml文件与下拉框的android:entries属性进行绑定,此绑定方式是静态的,不能根据其他控键的状态实时的改变下拉框的值。
-
首先选择工程中的values选项,右键
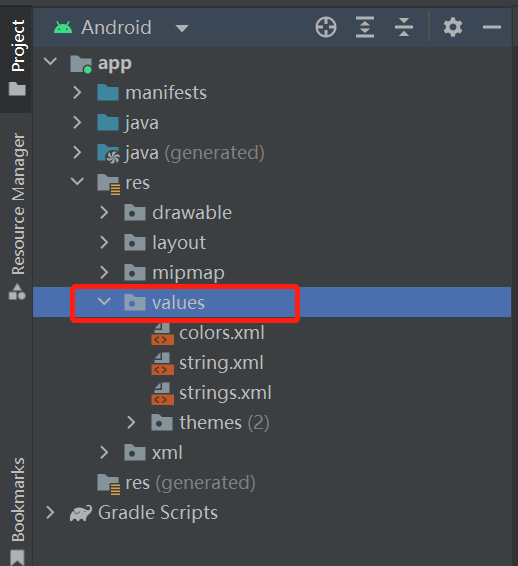
-
新建一个values xml资源文件
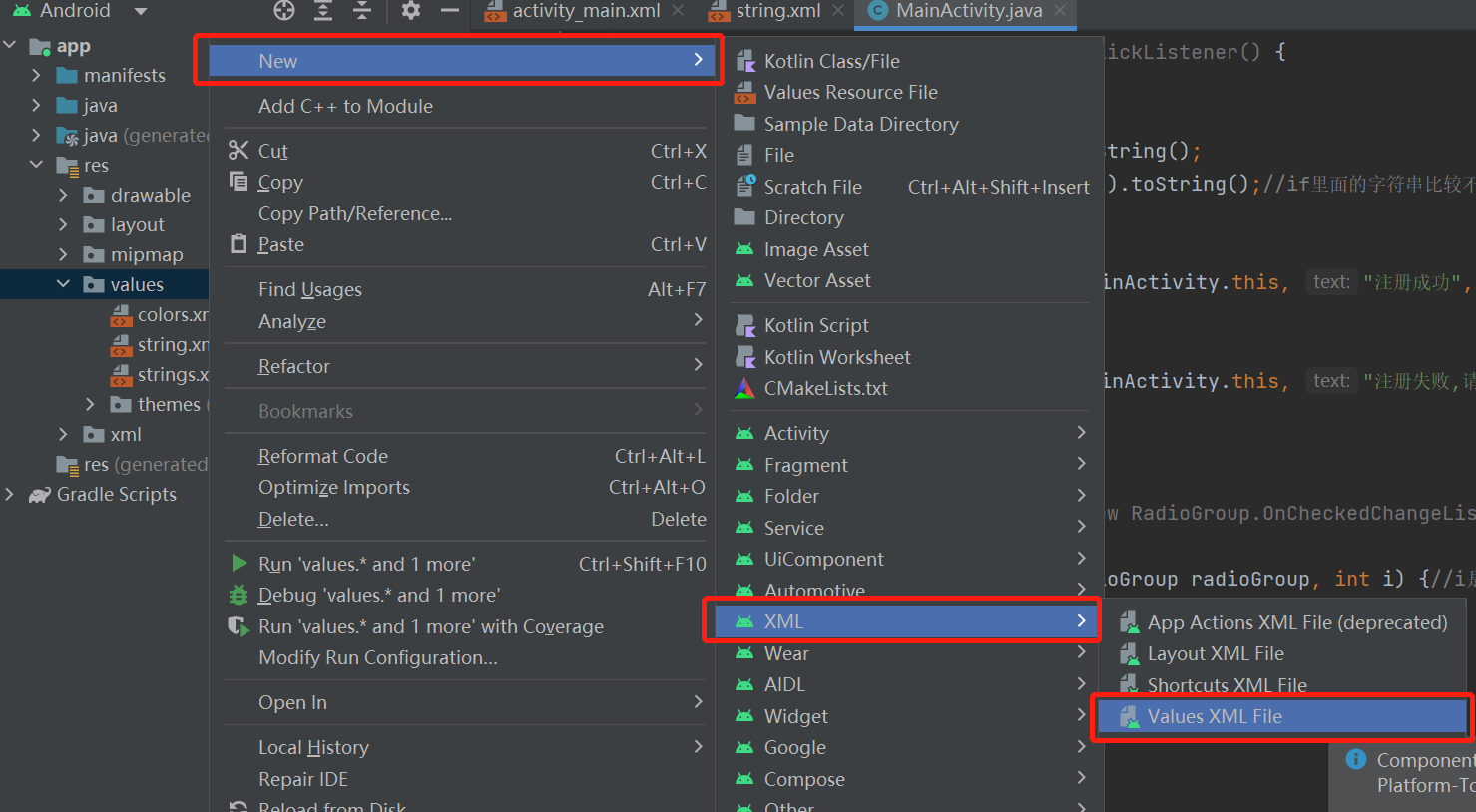
-
初始化一个数组为下拉列表绑定做好准备
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string-array name="wanggong">
<item>网工B20-1</item>
<item>网工B20-</item>
</string-array>
<string-array name="jike">
<item>计科B20-1</item>
<item>计科B20-</item>
</string-array>
<string-array name="ruangong">
<item>软工B20-1</item>
<item>软工B20-</item>
</string-array>
</resources>
-
在xml文件中找到下拉列表使用entries属性进行绑定指定的数组。
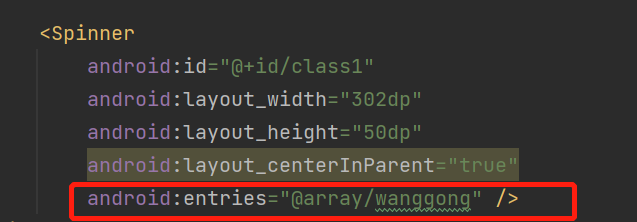
动态绑定
通过添加数组适配器与下拉框进行绑定,此绑定方式是动态的,只要在java文件中,根据逻辑需要修改数组适配器的值,再与下拉框进行绑定,就能根据其他控键的状态实时的改变下拉框的值。
在动态绑定之前,也是需要进行数据准备的,需要为适配器的实例化做准备。具体数据准备过程与静态绑定数据准备一样。
java文件:
package com.example.shiyan1_1;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.EditText;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.Spinner;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private RadioGroup subject;//单选按钮组,专业
private EditText pwd;//输入框,密码
private EditText pwdcheck;//输入框,确认密码
private Button sign;//按钮,注册
private Spinner class1;//下拉框,班级
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
subject = findViewById(R.id.subject);
//初始化各对象
pwd = findViewById(R.id.editTextTextPassword4);
pwdcheck = findViewById(R.id.editTextTextPasswordcheck);
sign = findViewById(R.id.sign);
class1 = findViewById(R.id.class1);
//按键响应(匿名内部类方式),当密码与确认密码一致时,在主界面显示注册成功,否则显示注册失败
sign.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
String p1 = pwd.getText().toString();//定义两个字符串变量进行储存控键的文本
String p2 = pwdcheck.getText().toString();//if里面的字符串比较不能用==,要使用equals。switch是支持字符串比较的
if (p1.equals(p2)) {
Toast.makeText(MainActivity.this, "注册成功", Toast.LENGTH_SHORT).show();
} else
Toast.makeText(MainActivity.this, "注册失败,请确认密码是否正确", Toast.LENGTH_SHORT).show();
}
});
//下拉框变化响应,当选中软工专业时,班级要进行相应的变化,变化为软工的班级
subject.setOnCheckedChangeListener(new RadioGroup.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(RadioGroup radioGroup, int i) {//i是选中按钮的id号
class1.setAdapter(radioButtonCheckded(i));//为下拉框绑定数组适配器
}
});
}
//自定义方法,对数组适配器按照程序逻辑进行不同的实例化,并返回适配器。
private ArrayAdapter radioButtonCheckded(int chackedRadioButtonId) {
RadioButton rb = findViewById(chackedRadioButtonId);//根据单选按钮组中的id号来确定初始化哪一个单选按钮组
String checked = rb.getText().toString();//定义数组来储存选中按钮的文本
ArrayAdapter<String> adapter=null;
if (checked.equals("网工"))
{
String[] wgclass = {"网工B20-1", "网工B20-2"};
//对数组适配器进行不同的实例化,第二个参数是显示样式
adapter = new ArrayAdapter<String>(MainActivity.this, androidx.appcompat.R.layout.support_simple_spinner_dropdown_item, wgclass);
}
if (checked.equals("计科"))
{
String[] jktclass = {"计科B20-1", "计科B20-2"};
adapter = new ArrayAdapter<String>(MainActivity.this, androidx.appcompat.R.layout.support_simple_spinner_dropdown_item, jktclass);
}
if (checked.equals("软工"))
{
String[] rgclass = {"软工B20-1", "软工B20-2"};
adapter = new ArrayAdapter<String>(MainActivity.this, androidx.appcompat.R.layout.support_simple_spinner_dropdown_item, rgclass);
}
return adapter;
}
}
布局文件:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity"
>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="35dp"
android:orientation="horizontal">
<TextView
android:id="@+id/textView6"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="请输入您的注册信息:" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="用户"
android:textSize="20sp" />
<EditText
android:id="@+id/user"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:ems="10"
android:hint="请输入用户名"
android:inputType="textPersonName"
android:textSize="20sp" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/textView4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="密码"
android:textSize="20sp" />
<EditText
android:id="@+id/editTextTextPassword4"
android:layout_width="236dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:ems="10"
android:inputType="textPassword"
android:minHeight="48dp"
android:hint="请输入6位密码"
tools:ignore="SpeakableTextPresentCheck" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/passwordcheck"
android:layout_width="38dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="确认密码"
android:textSize="20sp" />
<EditText
android:id="@+id/editTextTextPasswordcheck"
android:layout_width="236dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:ems="10"
android:inputType="textPassword"
android:minHeight="48dp"
android:hint="请确认密码"
tools:ignore="SpeakableTextPresentCheck" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/textView5"
android:layout_width="218dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="专业"
android:textSize="20sp" />
<RadioGroup
android:id="@+id/subject"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"
android:orientation="horizontal">
<RadioButton
android:id="@+id/wanggong"
android:layout_width="75dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:checked="true"
android:text=" 网工"
android:textSize="20sp"
tools:ignore="TouchTargetSizeCheck" />
<RadioButton
android:id="@+id/jike"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="计科"
android:textSize="20sp"
tools:ignore="TouchTargetSizeCheck" />
<RadioButton
android:id="@+id/ruangong"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="软工"
android:textSize="20sp"
tools:ignore="TouchTargetSizeCheck" />
</RadioGroup>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="45dp"
android:orientation="horizontal">
<TextView
android:id="@+id/textView7"
android:layout_width="149dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="班级"
android:textSize="20sp" />
<Spinner
android:id="@+id/class1"
android:layout_width="302dp"
android:layout_height="50dp"
android:layout_centerInParent="true"
android:entries="@array/wanggong" />
</LinearLayout>
<Button
android:id="@+id/sign"
android:layout_width="match_parent"
android:layout_height="62dp"
android:text="注册"
android:textColor="@color/black"
android:textSize="20dp" />
</LinearLayout>
数组资源文件:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string-array name="wanggong">
<item>网工B20-1</item>
<item>网工B20-</item>
</string-array>
<string-array name="jike">
<item>计科B20-1</item>
<item>计科B20-</item>
</string-array>
<string-array name="ruangong">
<item>软工B20-1</item>
<item>软工B20-</item>
</string-array>
</resources>
程序运行结果:
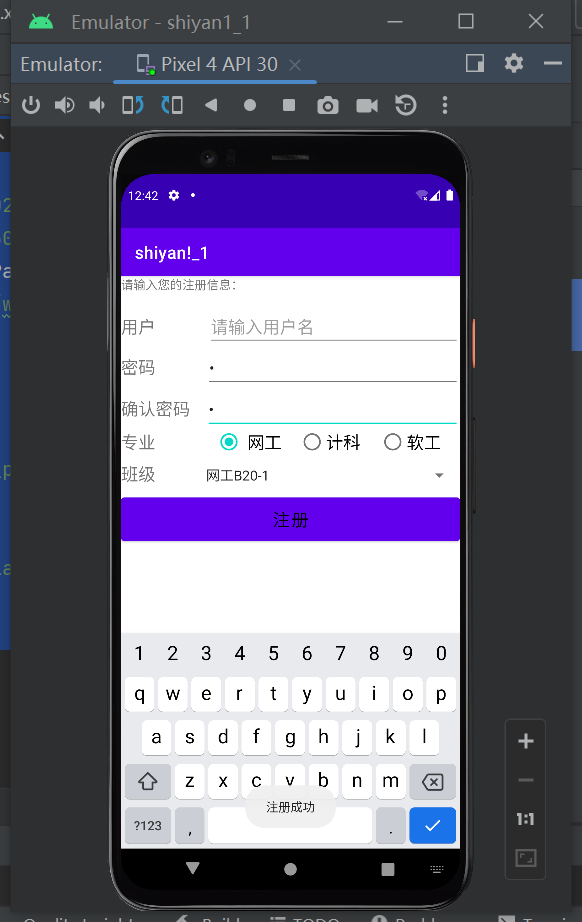
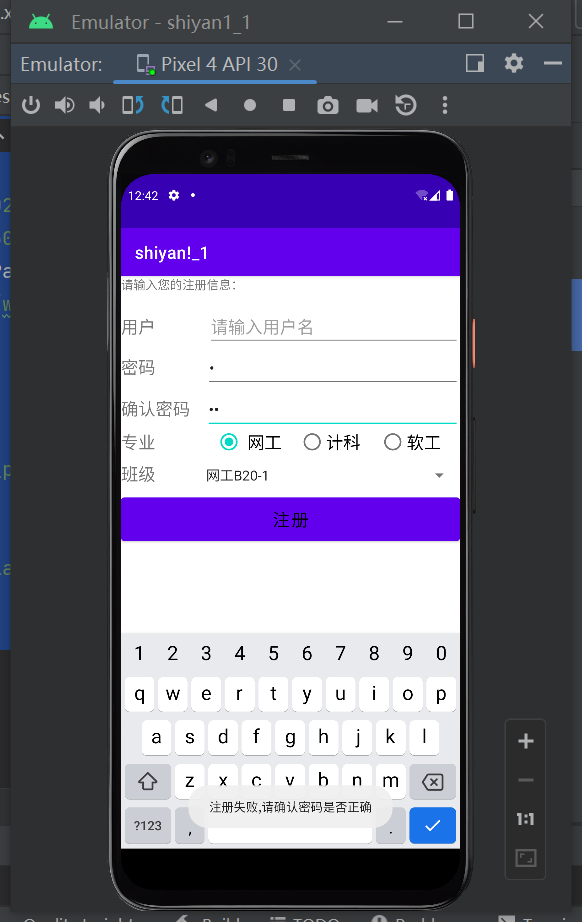
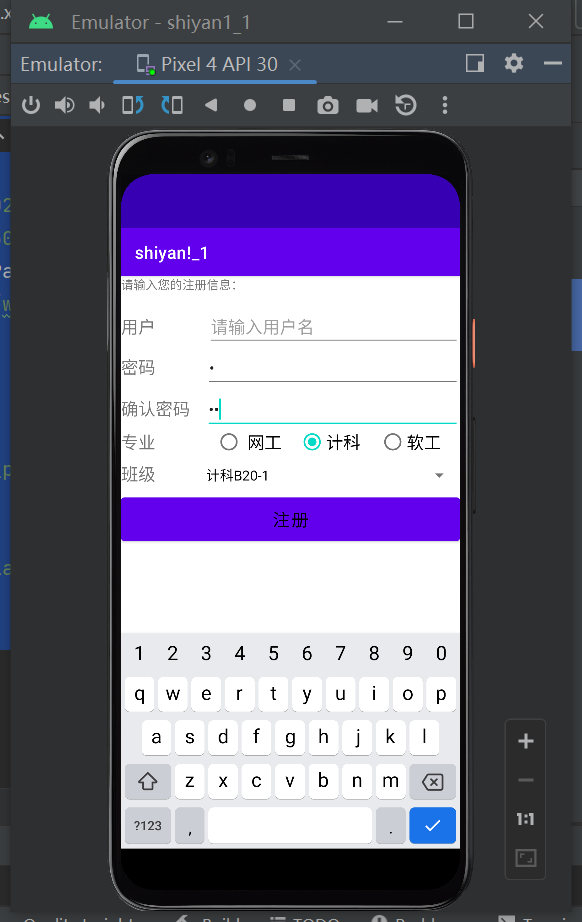
版权声明:本文为m0_58985552原创文章,遵循 CC 4.0 BY-SA 版权协议,转载请附上原文出处链接和本声明。