自定义异常
通常情况下,程序很少自行抛出异常,因为异常的类名通常也包含了该异常的有用信息。所以在抛出异常的时候应该选择合适的异常类。从而明确该异常情况。这些情况下需要自定义异常。
自定义异常的步骤如下:
-
1.继承RuntimeException 或者Exception
-
2.写一个无参的构造函数
-
3.写一个String类型的构造函数
public class SelfException extends RuntimeException{
/**
* 自定义异常类
* 1、继承RuntimeException或者Exception
* 2、写一个无参的构造函数
* 3、写一个String类型的构造函数
*/
public SelfException()
{
}
public SelfException(String msg)
{
super(msg);
}
}
public class SelfExceptionDemo {
//定义方法f1
public static double f1(int a,int b)
{
//求解两个数相除,要求b不能为负数,否则抛出异常
if(b<0)
throw new SelfException("被除数不能为负数!!");
else if(b==0)
throw new RuntimeException("被除数不能为0");
return a/b;
}
public static void main(String[] args) {
//测试
System.out.println(f1(2,5));
}
}
案例分析:模拟借书系统
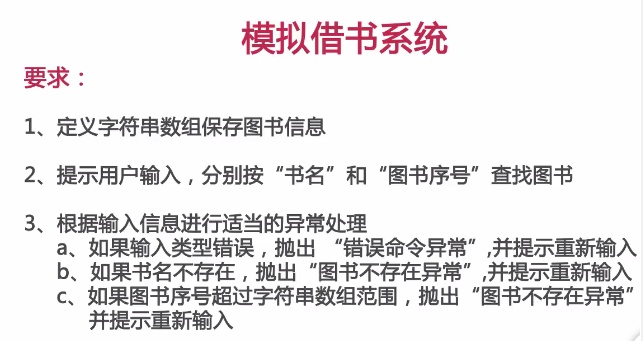
public class BorrowBookException extends Exception {
/**
* 自定义异常类
* 1、继承RuntimeException或者Exception
* 2、写一个无参的构造函数
* 3、写一个String类型的构造函数
*/
public BorrowBookException()
{
}
public BorrowBookException(String str)
{
super(str);
}
}
public class BorrowBookSystem {
public static LinkedHashMap save(String[] name,String[] id)
{
//1.根据定义字符串数组保存图书信息(书名、图书序号)
LinkedHashMap lhm = new LinkedHashMap();
for(int i=0;i<name.length;i++)
lhm.put(id[i], name[i]);
return lhm;
}
public static void main(String[] args) {
String[] name = {"java","语文","数学","英语","物理","化学","生物","政治","高数","Oracle"};
String[] id = {"1","2","3","4","5","6","7","8","9","10"};
Scanner sc = new Scanner(System.in);
LinkedHashMap lhm = save(name,id);
while(true)
{
System.out.println("请选择要进行查找的方式:1.书名 2.图书序号");
int choose = sc.nextInt();
if(!(choose==1||choose==2))
try {
throw new BorrowBookException("输入类型错误,请重新输入");
} catch (BorrowBookException e) {
System.out.println(e.toString());//输出异常提示信息
}
else if(choose==1)
{
System.out.println("请输入要查询的信息(输入exit退出)");
String str = sc.next();
if(!str.equals("exit"))
{
if(!lhm.containsValue(str))
try {
throw new BorrowBookException("图书不存在,请重新输入...");
} catch (BorrowBookException e) {
e.printStackTrace();//输出具体的异常信息
}
else
System.out.println("图书查找成功");
}
else
{
System.exit(0);
}
}
else if(choose==2)
{
System.out.println("请输入要查询的信息(输入exit退出)");
String str = sc.next();
if(!str.equals("exit"))
{
if(Integer.valueOf(str)>lhm.keySet().size())
try {
throw new BorrowBookException("图书不存在异常,请重新输入...");
} catch (BorrowBookException e) {
e.printStackTrace();
}
else
System.out.println("图书查找成功");
}
else
{
System.exit(0);
}
}
}
}
}
补充:java判断输入数据是否为纯数字?
-
使用Character.isDigit(char)判断
char num[] = str.toCharArray();//把字符串转换为字符数组
StringBuffer title = new StringBuffer();//用StringBuffer将非数字放入title中
StringBuffer hire = new StringBuffer();//把数字放到hire中
for (int i = 0; i < num.length; i++)
{
// 判断输入的数字是否为数字还是字符
if (Character.isDigit(num[i]))
{
/**
* 把字符串转换为字符,再调用Character.isDigit(char)
* 方法判断是否是数字,是返回True,否则False
*/
hire.append(num[i]);// 如果输入的是数字,把它赋给hire
}
else
{
title.append(num[i]);// 如果输入的是字符,把它赋给title
}
}
-
使用类型转换判断
try {
String str="123abc";
}
int num=Integer.valueOf(str);//把字符串强制转换为数字
return true;//如果是数字,返回True
} catch (Exception e) {
return false;//如果抛出异常,返回False
}
使用正则表达式判断
String str = "";
boolean isNum = str.matches("[0-9]+");
/**
* +表示1个或多个(如"3"或"225")
* *表示0个或多个([0-9]*)(如""或"1"或"22")
* ?表示0个或1个([0-9]?)(如""或"7")
* ps:这个方法只能用于判断是否是正整数
*/
版权声明:本文为weixin_39718083原创文章,遵循 CC 4.0 BY-SA 版权协议,转载请附上原文出处链接和本声明。