目
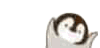
录
LocalDate、 LocalTime、 LocalDateTime类
DateTimeFormatter解析和格式化日期或时间的类
一.JDK1.7版本的新特性
1.二进制字面量
JDK7开始,可以
用二进制来表示整数
(byte,short,int和long)。使用二进制字面量的好处是可以使代码更容易被理解。语法非常简单,只要在二进制数值前面加 0b或者0B, 例: int x = 0b110110.
2.数字字面量可以出现下划线
为了增强对数值的阅读性,如我们经常把数据用逗号分隔一样。JDK7提供了
_对数据分隔
。注意事项:不能出现在进制标识和数值之间 / 不能出现在数值开头和结尾 / 不能出现在小数点旁边. 例:int x = 100_1000;
3.switch 语句可以用字符串
public class Test {
public static void main(String[] args) {
String s="HELLO";
switch (s){
case "a":
System.out.println("a");
break;
case "HELLO":
System.out.println("HELLO");
break;
}
}
}
还有一个泛型简化的特性
二.JDK1.8版本的新特性

1.新特性一:新增的日期时间相关类
LocalDate、 LocalTime、 LocalDateTime类

LocalDate、 LocalTime、 LocalDateTime类的实例是不可变的对象, 分别表示使用 ISO-8601日历系统的
日期、时间、日期和时间
。 它们提供了简单的日期或时间,并不包含当前的时间信息。也不包含与时区相关的信息。 注: ISO-8601日历系统是国际标准化组织制定的现代公民的日期和时间的表示法 这些新增的日期时间API都在 java.time包下
获取对象的方法
-
方式1通过静态方法 now(); 例如:LocalDateTime ldt = LocalDateTime.now();
-
方式2通过静态方法of()方法参数可以指定年月日时分秒; 例如:LocalDateTime of =LocalDateTime.of(2018, 12, 30, 20, 20, 20);
常用方法
-
ldt.getYear(); //获取年
-
ldt.getMinute(); //获取分钟
-
ldt.getHour(); //获取小时
-
getDayOfMonth //获得月份天数(1-31)
-
getDayOfYear //获得年份天数(1-366)
-
getDayOfWeek //获得星期几(返回一个 DayOfWeek枚举值)
-
getMonth //获得月份, 返回一个 Month 枚举值
-
getMonthValue //获得月份(1-12)
-
getYear //获得年份
-
format() //格式化日期日期字符串的方法 例如:String yyyy = ldt.format(DateTimeFormatter.ofPattern(“yyyy”));
-
toLocalDate(); / toLocalTime(); //转换的方法 例如:LocalDate localDate = ldt.toLocalDate(); 例如:LocalTime localTime = ldt.toLocalTime();
-
isAfter() //判断一个日期是否在指定日期之后 例如: boolean after = ldt.isAfter(LocalDateTime.of(2024, 1, 1, 2, 3));
-
isBefore() //判断一个日期是否在指定日期之前
-
isEqual() //判断两个日期是否相同
-
isLeapYear() //判断是否是闰年注意是LocalDate类中的方法 例如 boolean b= LocalDate.now().isLeapYear();
-
parse(“2007-12-03T10:15:30”); //将一个日期字符串解析成日期对象,注意字符串日期的写法的格式要正确,否则解析失败. 注意细节:如果用LocalDateTime 想按照我们的自定义的格式去解析,注意日期字符串的 年月日时分秒要写全,不然就会报错 例如:LocalDateTime parse = LocalDateTime.parse(“2007-12-03T10:15:30”);
-
plusYears(); / plusMonths(); / ……. //添加年月日时分秒的方法 plus系列的方法 都会返回一个新的LocalDateTime的对象 例如:LocalDateTime localDateTime = ldt.plusYears(1);
-
minusYears(); / minusMonths(); / ……. //减去年月日时分秒的方法 minus 系列的方法 注意都会返回一个新的LocalDateTime的对象 例如:LocalDateTime localDateTime2 = ldt.minusYears(8);
-
withYears(); / withMonths(); / ……. //指定年月日时分秒的方法 with系列的方法 注意都会返回一个新的LocalDateTime的对象 例如 LocalDateTime localDateTime3 = ldt.withYear(1998);
Instant 时间戳类

-
Instant ins = Instant.now(); //获取对象的方法 now(), 注意默认获取出来的是当前的美国时间和我们的北京时间相差八个小时
-
atOffset() //设置偏移量 例如:OffsetDateTime time = ins.atOffset(ZoneOffset.ofHours(8));
-
ZoneId.systemDefault() //获取本地的默认时区ID
-
atZone() //获取系统默认时区时间的方法,其参数是要一个时区的编号, 可以通过时区编号类获取出来 例如: ZonedDateTime zonedDateTime = ins.atZone(ZoneId.systemDefault());
-
getEpochSecond() //获取从1970-01-01 00:00:00到当前时间的秒值 例如:long epochSecond = ins.getEpochSecond();//获取从1970-01-01 00:00:00到当前时间的秒值
-
toEpochMilli() //获取从1970-01-01 00:00:00到当前时间的毫秒值
-
getNano() //方法是把获取到的当前时间的秒数 换算成纳秒 例如:当前时间是2018-01-01 14:00:20:30 , 那就把30豪秒换算成纳秒 int nano = ins.getNano()
-
ofEpochSecond() //给计算机元年增加秒数 例如:Instant instant = Instant.ofEpochSecond(5);
-
ofEpochMilli() //给计算机元年增加毫秒数 补充:单位换算 0.1 毫秒 = 10 的5次方纳秒 = 100000 纳秒 1 毫秒 = 1000 微妙 = 1000000 纳秒
Duration类和Period类

Duration
是用于
计算两个“时间”间隔
的类, 而
Period
是用于
计算两个“日期”间隔
的类 .
Duration类常用的方法如下:
-
between() //计算两个时间的间隔,默认是秒 例如:Duration between = Duration.between(start, end);
-
toMillis() //将秒转成毫秒
Period类计算两个日期之间的间隔的代码如下:
LocalDate s = LocalDate.of(1985, 03, 05);
LocalDate now = LocalDate.now();
Period be = Period.between(s, now);
System.out.println(be.getYears());间隔了多少年
System.out.println(be.getMonths());间隔了多少月
System.out.println(be.getDays());间隔多少天
TemporalAdjuster时间矫正器

TemporalAdjuster时间矫正器是一个接口,一般我们用该接口的一个对应的工具类, TemporalAdjusters中的一些常量,来指定日期
LocalDate now = LocalDate.now();
System.out.println(now);
//1 使用TemporalAdjusters自带的常量来设置日期
LocalDate with = now.with(TemporalAdjusters.lastDayOfYear());
System.out.println(with);
//2 采用TemporalAdjusters中的next方法来指定日期
LocalDate date = now.with(TemporalAdjusters.next(DayOfWeek.SUNDAY));
System.out.println(date);
例如:TemporalAdjusters.next(DayOfWeek.SUNDAY) 本周的星期天
例如:TemporalAdjusters.nextOrSame(DayOfWeek.MONDAY) 下一周的星期一
//3 采用自定义的方式来指定日期 比如指定下个工作日
LocalDateTime ldt = LocalDateTime.now();
LocalDateTime workDay = ldt.with(new TemporalAdjuster() {
@Override
public Temporal adjustInto(Temporal temporal) {
//向下转型
LocalDateTime ld = (LocalDateTime) temporal;
//获取这周的星期几
DayOfWeek dayOfWeek = ld.getDayOfWeek();
if (dayOfWeek.equals(DayOfWeek.FRIDAY)) {
return ld.plusDays(3);//如果这天是星期五,那下个工做日就加3天
} else if (dayOfWeek.equals(DayOfWeek.SATURDAY)) {
return ld.plusDays(2);//如果这天是星期六,那下个工做日就加2天
} else {
//其他就加一天
return ld.plusDays(1);
}
}
});
System.out.println(workDay);
DateTimeFormatter解析和格式化日期或时间的类

常用的方法:
-
获取对象的方式,通过静态方法ofPattern(“yyyy-MM-dd”);
DateTimeFormatter dateFormat = DateTimeFormatter.ofPattern("yyyy-MM-dd");
LocalDateTime now = LocalDateTime.now();
-
format()方法把一个日期对象的默认格式 格式化成指定的格式
String format1 = dateFormat.format(now);
System.out.println(format1);
-
格式化日期 方式2使用日期类中的format方法 传入一个日期格式化类对象
LocalDateTime now1 = LocalDateTime.now();
//使用日期类中的format方法 传入一个日期格式化类对象
//使用DateTimeFormatter中提供好的日期格式常量
now1.format(DateTimeFormatter.ISO_LOCAL_DATE_TIME);
-
使用自定义的日期格式格式化字符串
DateTimeFormatter timeFormat = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");//自定义一个日期格式
String time = now1.format(timeFormat);
System.out.println(time);
-
把一个日期字符串转成日期对象, 使用日期类中的parse方法传入一个日期字符串,传入对应的日期格式化类
DateTimeFormatter dateFormat = DateTimeFormatter.ofPattern("yyyy-MM-dd");
LocalDateTime parse = LocalDateTime.parse(time, timeFormat);
System.out.println(parse);
补:
ZonedDate,ZonedTime和ZonedDateTime : 带时区的时间或日期, 用法和 LocalDate、 LocalTime、 LocalDateTime 一样 只不过ZonedDate,ZonedTime、ZonedDateTime 这三个带有当前系统的默认时区
ZoneID 世界时区类

-
getAvailableZoneIds() //获取世界各个地方的时区的集合, 使用ZoneID中的静态方法getAvailableZoneIds()来获取 例如:Set<String> availableZoneIds = ZoneId.getAvailableZoneIds();
-
systemDefault() //获取系统默认时区的ID
-
获取带有时区的日期时间对象
//创建日期对象
LocalDateTime now = LocalDateTime.now();
//获取不同国家的日期时间根据各个地区的时区ID名创建对象
ZoneId timeID = ZoneId.of("Asia/Shanghai");
//根据时区ID获取带有时区的日期时间对象
ZonedDateTime time = now.atZone(timeID);
System.out.println(time);
//方式2 通过时区ID 获取日期对象
LocalDateTime now2 = LocalDateTime.now(ZoneId.of("Asia/Shanghai"));
System.out.println(now2);

2.特性二:Lambda表达式
Lambda 是一个匿名函数,我们可以把 Lambda表达式理解为是一段可以传递的代码(将代码像数据一样进行传递)。可以写出更简洁、更灵活的代码。作为一种更紧凑的代码风格,使Java的语言表达能力得到了提升。也就相当于一种简写模式!
Lambda表达式的书写语法

Lambda 表达式在Java 语言中引入了一个新的语法元素和操作符。这个操作符为 “ ->” , 该操作符被称为 Lambda 操作符或箭头操作符。它将 Lambda 分为两个部分:左侧和右侧!
左侧: 指定了 Lambda 表达式需要的所有参数(形参列表) 右侧: 指定了 Lambda 体,即 Lambda 表达式要执行的功能
。(方法的具体实现)例:
public static void main(String[] args) {
//匿名内部类
MyInterface myInterface = new MyInterface() {
@Override
public void show() {
System.out.println("哈哈");
}
};
//lambda表达式
MyInterface myInterface2 = () -> {
System.out.println("哈哈");
};
匿名内部类的Lambda表达式简化规则

-
◾ 左边部分:是形参列表 右边部分:是对接口的抽象方法的具体实现 同时可以
省略形参类型
-
◾ 如果对接中方法的实现,
只有一行代码,那么{}和return都可以省略不写
, 反之不能省略
-
◾ 如果
形参只有一个,形参的括号可以省略
不写
MyInterface myInterface = new MyInterface() {
@Override
public Integer show(Integer a, Integer b) {
return a + b;
}
};
//第一步:简化 左边部分:是形参列表 右边部分:是对接口的抽象方法的具体实现
MyInterface myInterface2 = (Integer a, Integer b) -> {
return a + b;
};
//第二步简化:可以省略形参类型
MyInterface myInterface3 = (a, b) -> {
return a + b;
};
//第三步简化:对接中的方法的实现只有一行代码,那么{}和return都可以省略不写
MyInterface myInterface4 = (a, b) -> a + b;
//如果对接中的方法的实现,不止有一行代码,那么{}和return 不能省略
MyInterface myInterface = new MyInterface() {
@Override
public Integer show(Integer a, Integer b) {
a += 10;
b += 20;
return a + b;
}
};
//省略后
MyInterface myInterface5 = (a, b) -> {
a += 10;
b += 20;
return a + b;
};
//如果 形参只有一个,形参的括号可以省略不写
AA aa = a -> System.out.println(a);
}
}
Lambda表达式可作为参数传递

Lambda表达式,
只支持函数式接口
。函数式接口就是这个接口中只有一个抽象方法。
@FunctionalInterface 这个注解可以标识他是一个函数式接口
。我们可以在任意函数式接口上使用 @FunctionalInterface 注解,这样做可以检查它是否是一个函数式接口。
@FunctionalInterface //这个注解可以标识他是一个函数式接口
//函数式接口
public interface MyInterface {
Integer show(Integer a, Integer b);
}
public class MyTest {
public static void main(String[] args) {
Integer[] arr = {20, 1, 6};
//给数组arr排序
Arrays.sort(arr, new Comparator<Integer>() {
@Override
public int compare(Integer x, Integer y) {
return x - y;
}
});
//Lambda表达式 作为参数传递
Comparator<Integer> tComparator = (a, b) -> a - b;
Arrays.sort(arr, tComparator);
Arrays.sort(arr, (a, b) -> a - b);
}
}
Java中提供的4大核心函数式接口
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Lambda表达式的另一种写法, 方法引用

方法引用其实是Lambda表达式的另一种写法, 当要传递给Lambda体的操作,已经有实现的方法了,可以使用方法引用. 注意:实现抽象方法的参数列表,必须与方法引用方法的参数列表保持一致!方法引用:使用操作符 “ ::” 将方法名和对象或类的名字分隔开来。存在三种主要使用情况,对象::实例方法 / 类::静态方法 / 类::实例方法
public class MyTest {
public static void main(String[] args) {
//情况一 对象::实例方法
Consumer<String> consumer = new Consumer<String>() {
@Override
public void accept(String s) {
PrintStream out = System.out;
out.println(s);
}
};
Consumer<String> consumer2 = msg -> System.out.println(msg);
//最终版的简化
Consumer<String> consumer3 = System.out::println;
//情况二 类::静态方法
int max = Math.max(5, 6);
BiFunction<Integer, Integer, Integer> biFunction = new BiFunction<Integer, Integer, Integer>() {
@Override
public Integer apply(Integer x, Integer y) {
return Math.max(x, y);
}
};
BiFunction<Integer, Integer, Integer> biFunction2 = (x, y) -> Math.max(x, y);
//最终简化
BiFunction<Integer, Integer, Integer> biFunction3 = Math::max;
//情况三 类::实例方法
Comparator<String> comparator = new Comparator<String>() {
@Override
public int compare(String x, String y) {
return x.compareTo(y);
}
};
Comparator<String> comparator2 = (x, y) -> x.compareTo(y);
//最终简化
Comparator<String> comparator3 = String::compareTo;
}
}
构造器引用

构造器引用的
格式:ClassName::new。与函数式接口相结合,自动与函数式接口中方法兼容。可以把构造器引用赋值给定义的方法,与构造器参数列表要与接口中抽象方法的参数列表一致!
public class Student {
String name;
int age;
public Student() {
}
public Student(String name, int age) {
this.name = name;
this.age = age;
}
}
public class MyTest {
public static void main(String[] args) {
Supplier<Student> supplier = new Supplier<Student>() {
@Override
public Student get() {
return new Student();
}
};
Supplier<Student> supplier2 = () -> new Student();
//最终简化
Supplier<Student> supplier3 = Student::new;
BiFunction<String, Integer, Student> biFunction = new BiFunction<String, Integer, Student>() {
@Override
public Student apply(String name, Integer age) {
return new Student(name, age);
}
};
BiFunction<String, Integer, Student> biFunction2 = (name, age) -> new Student(name, age);
//最终简化
BiFunction<String, Integer, Student> biFunction3 = Student::new;
}
}

3 .特性三:Stream流
Stream 是 Java8 中处理集合的关键抽象概念, 它可以指定你希望对集合进行的操作, 可以执行非常复杂的查找、过滤和映射数据等操作。 使用Stream API 对集合数据进行操作,就类似于使用 SQL 执行的数据库查询。也可以使用 Stream API 来并行执行操作。 简而言之,
Stream API 提供了一种高效且易于使用的处理数据的方式。
流(Stream)是数据渠道,用于操作数据源(集合、数组等)所生成的元素序列。 集合讲的是数据,流讲的是计算! 需要注意:
-
Stream 自己不会存储元素。
-
Stream 不会改变源对象。相反,他们会返回一个持有结果的新Stream。
-
Stream 操作是延迟执行的。这意味着他们会等到需要结果的时候才执行。
Stream 的操作三个步骤

-
创建 Stream : 一个数据源(如:集合、数组),获取一个流
-
中间操作 : 一个中间操作链,对数据源的数据进行处理
-
终止操作(终端操作) : 一个终止操作,执行中间操作链,并产生结果
创建Stream的方式

? Java8 中的 Collection 接口被扩展,提供了两个获取流的方法:
-
default Stream<E> stream() 返回一个顺序流
-
default Stream<E> parallelStream() 返回一个并行流
? Java8 中的 Arrays 的静态方法 stream() 可以获取数组流:static <T> Stream<T> stream(T[] array): 返回一个流重载形式,能够处理对应基本类型的数组:
-
public static IntStream stream(int[] array)
-
public static LongStream stream(long[] array)
-
public static DoubleStream stream(double[] array)
? 由值创建流,可以使用静态方法 Stream.of(), 通过显示值创建一个流。它可以接收任意数量的参数。
-
public static<T> Stream<T> of(T… values) 返回一个流
? 由函数创建流:创建无限流可以使用静态方法 Stream.iterate()和Stream.generate(), 创建无限流。
-
public static<T> Stream<T> iterate(final T seed, finalUnaryOperator<T> f) 迭代
-
public static<T> Stream<T> generate(Supplier<T> s) 生成
public class MyTest {
public static void main(String[] args) {
//创建流
//方式1:
List<Integer> list = Arrays.asList(100, 200, 300, 7000, 90, 4);
//集合里面有一个 stream() 方法,就可以得到这个流
Stream<Integer> stream = list.stream();
Integer[] arr = {20, 30, 80, 35, 85};
//方式2
//Arrays里面的静态方法stream()方法,就可以得到这个流
Stream<Integer> stream1 = Arrays.stream(arr);
//方式3 Stream类他有一个静态方法,可以得到这个流
Stream<Integer> stream2 = Stream.of(100, 200, 300, 7000, 90, 100);
//方式4 创建无限流
Stream<Double> generate = Stream.generate(Math::random);
//方式5:创建无限流
Stream<Integer> iterate = Stream.iterate(1, new UnaryOperator<Integer>() {
@Override
public Integer apply(Integer integer) {
return integer + 1;
}
});
iterate.limit(10).forEach(System.out::println);
}
}
Stream 的中间操作

多个中间操作可以连接起来形成一个流水线,除非流水线上触发终止操作,否则中间操作不会执行任何的处理!而在终止操作时一次性全部处理,称为“
惰性求值
”。
?
筛选与切片
-
filter(Predicate p) 过滤 接收 Lambda , 从流中排除某些元素。
-
distinct() 去重,通过流所生成元素的 hashCode() 和 equals() 去除重复元素
-
limit(long maxSize) 截断流,使其元素不超过给定数量。
-
skip(long n) 跳过元素,返回一个扔掉了前 n 个元素的流。若流中元素不足 n 个,则返回一个空流。与 limit(n) 互补
?
映射
-
map(Function f) 接收一个函数作为参数,该函数会被应用到每个元素上,并将其映射成一个新的元素。
-
mapToDouble(ToDoubleFunction f) 接收一个函数作为参数,该函数会被应用到每个元素上,产生一个新的 DoubleStream。
-
mapToInt(ToIntFunction f) 接收一个函数作为参数,该函数会被应用到每个元素上,产生一个新的 IntStream。
-
mapToLong(ToLongFunction f) 接收一个函数作为参数,该函数会被应用到每个元素上,产生一个新的 LongStream。
?
排序
-
sorted() 产生一个新流,其中按自然顺序排序 元素实现Compareble接口
-
sorted(Comparator comp) 产生一个新流,其中按比较器顺序排序 传入一个比较
Stream 的终止操作

终端操作会从流的流水线生成结果。其结果可以是任何不是流的值,例如:List、Integer,甚至是 void 。
?
查找与匹配
-
allMatch(Predicate p) 检查是否匹配所有元素 比如判断 所有员工的年龄都是17岁 如果有一个不是,就返回false
-
anyMatch(Predicate p) 检查是否至少匹配一个元素 比如判断是否有姓王的员工,如果至少有一个就返回true
-
noneMatch(Predicate p) 检查是否没有匹配所有元素 employee.getSalary() < 3000; 每个员工的工资如果都高于3000就返回true 如果有一个低于3000 就返回false
-
findFirst() 返回第一个元素 比如获取工资最高的人 或者 获取工资最高的值是
-
findAny() 返回当前流中的任意元素 比如随便获取一个姓王的员工
-
count() 返回流中元素总数
-
max(Comparator c) 返回流中最大值 比如:获取最大年龄值
-
min(Comparator c) 返回流中最小值 比如:获取最小年龄的值
-
forEach(Consumer c) 内部迭代(使用 Collection 接口需要用户去做迭代,称为外部迭代。相反,Stream API 使用内部迭代——它帮你把迭代做了)
?
归约
-
reduce(T iden, BinaryOperator b) 参1 是起始值, 参2 二元运算 , 可以将流中元素反复结合起来,得到一个值。返回 T 比如: 求集合中元素的累加总和
-
reduce(BinaryOperator b) 这个方法没有起始值 可以将流中元素反复结合起来,得到一个值。返回 Optional<T> , 比如你可以算所有员工工资的总和
备注:map 和 reduce 的连接通常称为 map-reduce 模式,因 Google 用它来进行网络搜索而出名。
?
收集
-
collect(Collector c) 将流转换为其他形式。接收一个 Collector接口的实现,用于给Stream中元素做汇总的方法
public class MyTest {
public static void main(String[] args) {
//终止操作
List<Employee> list = Arrays.asList(
new Employee(101, "张三", 18, 9999.99),
new Employee(101, "张三", 18, 9999.99),
new Employee(101, "张三", 18, 9999.99),
new Employee(101, "张三", 18, 9999.99),
new Employee(103, "王五", 28, 3333.33),
new Employee(102, "李四", 59, 6666.66),
new Employee(104, "赵六", 80, 7777.77),
new Employee(104, "赵六", 80, 7777.77),
new Employee(104, "赵六", 80, 7777.77),
new Employee(105, "田七", 38, 5555.55)
);
//判断是否所有员工都满18岁了
//allMatch(Predicate p) 检查是否匹配所有元素
boolean b = list.stream().allMatch(emp -> emp.getAge() >= 18);
System.out.println(b);
//判断员工有么有姓王的
boolean b1 = list.stream().anyMatch(ele -> ele.getName().startsWith("刘"));
System.out.println(b1);
//是不是员工没有姓王
boolean b2 = list.stream().noneMatch(ele -> ele.getName().startsWith("王"));
System.out.println(b2);
//获取工资最高的员工
Optional<Employee> first = list.stream().sorted((x, y) -> (int) (y.getSalary() - x.getSalary())).findFirst();
Employee employee = first.get();
System.out.println(employee);
//获取最高工资
//findFirst() 返回第一个元素
Optional<Double> first1 = list.stream().map(Employee::getSalary).sorted((x, y) -> (int) (y - x)).findFirst();
Double aDouble = first1.get();
System.out.println(aDouble);
Employee employee = list.stream().max((a, b) -> (int) (a.getSalary() - b.getSalary())).get();
System.out.println(employee);
//要最小工资
Double aDouble = list.stream().map(Employee::getSalary).min((a, b) -> (int) (a - b)).get();
System.out.println(aDouble);
//统计总工资
Optional<Integer> reduce = list.stream().map(Employee::getAge).distinct().reduce((a, b) -> a + b);
Integer sum = reduce.get();
System.out.println(sum);
System.out.println("=============================");
Integer reduce1 = list.stream().distinct().map(Employee::getAge).reduce(0, (a, b) -> a + b);
System.out.println(reduce1);
//串行流 stream()
//并行流: parallelStream()
Optional<Employee> any = list.parallelStream().distinct().findAny();
Employee employee1 = any.get();
System.out.println(employee1);
long count = list.stream().distinct().count();
System.out.println(count);
}
}
?
Collectors 中的方法
Collector 接口中方法的实现决定了如何对流执行收集操作(如收集到 List、Set、Map)。但是 Collectors 实用类提供了很多静态方法,可以方便地创建常见收集器实例,具体方法与实例如下
-
List<T> toList() 把流中元素收集到List 比如把所有员工的名字通过map()方法提取出来之后,在放到List集合中去. 例子:List<Employee> emps= list.stream().map(提取名字).collect(Collectors.toList());
-
Set<T> toSet() 把流中元素收集到Set 比如把所有员工的名字通过map()方法提取出来之后,在放到Set集合中去. 例子:Set<Employee> emps= list.stream().collect(Collectors.toSet());
-
Collection<T> toCollection() 把流中元素收集到创建的集合 比如把所有员工的名字通过map()方法提取出来之后,在放到自己指定的集合中去. 例子:Collection<Employee>emps=list.stream().map(提取名字).collect(Collectors.toCollection(ArrayList::new));
-
Long counting() 计算流中元素的个数. 例子:long count = list.stream().collect(Collectors.counting());
-
Integer summingInt() 对流中元素的整数属性求和. 例子:inttotal=list.stream().collect(Collectors.summingInt(Employee::getSalary));
-
Double averagingInt() 计算流中元素Integer属性的平均值. 例子:doubleavg= list.stream().collect(Collectors.averagingInt(Employee::getSalary));
-
IntSummaryStatistics summarizingInt() 收集流中Integer属性的统计值。例子:DoubleSummaryStatistics dss= list.stream().collect(Collectors.summarizingDouble(Employee::getSalary));从DoubleSummaryStatistics 中可以获取最大值,平均值等double average = dss.getAverage();long count = dss.getCount();double max = dss.getMax();
-
String joining() 连接流中每个字符串 比如把所有人的名字提取出来,在通过”-“横杠拼接起来. 例子:String str= list.stream().map(Employee::getName).collect(Collectors.joining(“-“));
-
Optional<T> maxBy() 根据比较器选择最大值 比如求最大工资. 例子:Optional<Double> collect1 = list.stream().map(Employee::getSalary).collect(Collectors.maxBy((x, y) -> (int) (x – y)));
-
Optional<T> minBy() 根据比较器选择最小值 比如求最小工资. 例子: Optional<Double> collect1 = list.stream().map(Employee::getSalary).collect(Collectors.minBy((x, y) -> (int) (x – y)));
-
归约产生的类型 reducing() 从一个作为累加器的初始值开始,利用BinaryOperator与流中元素逐个结合,从而归约成单个值. 注意:把工资要提取出来,在进行 reducing(). 例子:Double collect = list.stream().map(Employee::getSalary).collect(Collectors.reducing(0.0, (x, y) -> x + y));
-
转换函数返回的类型 collectingAndThen() 包裹另一个收集器,对其结果转换函数. 例子:inthow= list.stream().collect(Collectors.collectingAndThen(Collectors.toList(), List::size));
-
Map<K, List<T>> groupingBy() 根据某属性值对流分组,属性为K,结果为V 比如按照 状态分组. 例子:Map<Emp.Status, List<Emp>> map= list.stream().collect(Collectors.groupingBy(Employee::getStatus));
-
Map<Boolean, List<T>> partitioningBy() 根据true或false进行分区 比如 工资大于等于6000的一个区,小于6000的一个区. 例子:Map<Boolean,List<Emp>>vd= list.stream().collect(Collectors.partitioningBy(Employee::getSalary));
public class MyTest {
public static void main(String[] args) {
List<Employee> list = Arrays.asList(
new Employee(102, "李四", 59, 6666.66),
new Employee(101, "张三", 18, 9999.99),
new Employee(103, "王五", 28, 3333.33),
new Employee(104, "赵六", 8, 7777.77),
new Employee(104, "赵六", 8, 7777.77),
new Employee(104, "赵六", 8, 7777.77),
new Employee(105, "田七", 38, 5555.55)
);
//把员工的姓名收集到一个新集合
//Collectors 他里面有很多的方法
List<String> collect = list.stream().distinct().map(Employee::getName).collect(Collectors.toList());
System.out.println(collect);
Set<String> collect1 = list.stream().map(Employee::getName).collect(Collectors.toSet());
System.out.println(collect1);
//可以收集到指定的集合
LinkedHashSet<String> collect2 = list.stream().map(Employee::getName).collect(Collectors.toCollection(LinkedHashSet::new));
System.out.println(collect2);
Long collect3 = list.stream().distinct().collect(Collectors.counting());
System.out.println(collect3);
Integer collect4 = list.stream().distinct().collect(Collectors.summingInt(Employee::getAge));
System.out.println(collect4);
System.out.println("===================================");
Double collect5 = list.stream().collect(Collectors.averagingDouble(Employee::getSalary));
System.out.println(collect5);
System.out.println("============================================");
DoubleSummaryStatistics dss = list.stream().collect(Collectors.summarizingDouble(Employee::getSalary));
double max = dss.getMax();
double min = dss.getMin();
long count = dss.getCount();
double sum = dss.getSum();
double average = dss.getAverage();
System.out.println(max);
System.out.println(min);
System.out.println(count);
System.out.println(average);
System.out.println(sum);
System.out.println("==========================================");
String collect6 = list.stream().map(Employee::getName).collect(Collectors.joining("-"));
System.out.println(collect6);
String collect7 = list.stream().map(Employee::getName).collect(Collectors.joining("-", "[", "]"));
System.out.println(collect7);
}
}
补:并行流stream()与串行流 parallelStream(), 并行流就是把一个内容分成多个数据块,并用不同的线程分别处理每个数据块的流。Java 8 中将并行进行了优化,我们可以很容易的对数据进行并行操作。Stream API 可以声明性地通过 parallel() 与sequential() 在并行流与顺序流之间进行切换。
(小编也在努力学习更多哟!以后再慢慢分享的啦!)
希望对友友们有所帮助!!!!