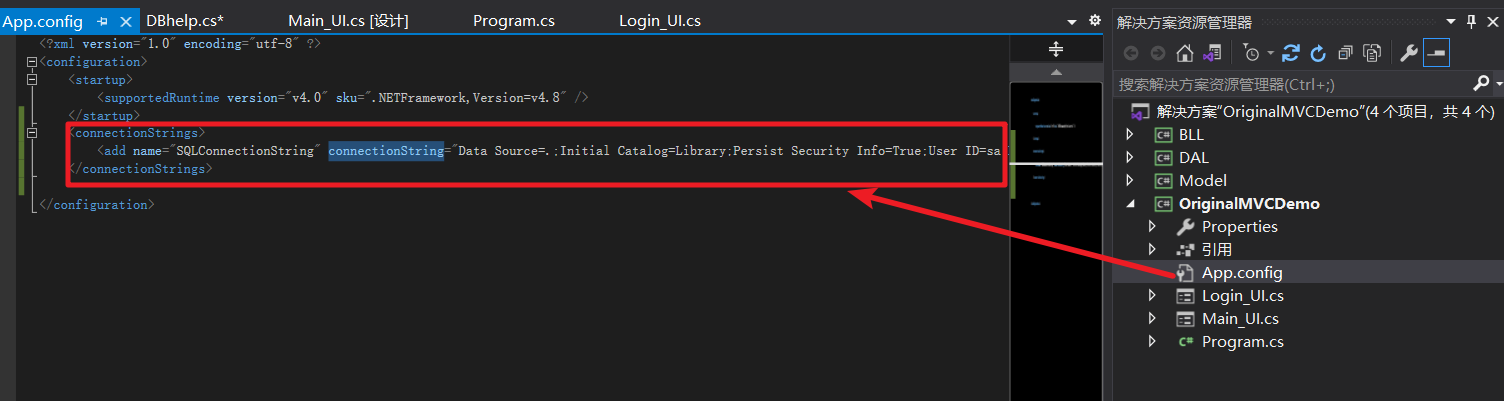
<connectionStrings>
<add name="SQLConnectionString" connectionString="Data Source=.;Initial Catalog=Library;Persist Security Info=True;User ID=sa;Password=666666"/>
</connectionStrings>
public class DBhelp
{
private DBhelp() { }
private static DBhelp dbhelp = null;
public static DBhelp Create()
{
if (dbhelp == null)
dbhelp = new DBhelp();
return dbhelp;
}
string conString = ConfigurationManager.ConnectionStrings["SQLConnectionString"].ConnectionString;
//返回一行一列 登录
public int ExecuteScalar(string sql, params SqlParameter[] sp)
{
SqlConnection con = new SqlConnection(conString);
try
{
con.Open();
SqlCommand com = new SqlCommand(sql, con);
com.Parameters.AddRange(sp);
return (int)com.ExecuteScalar();
}
catch (Exception)
{
con.Close();
throw;
}
finally
{
con.Close();
}
}
//返回读取器对象 需自行遍历
public SqlDataReader ExecuteReader(string sql, params SqlParameter[] sp)
{
SqlConnection con = new SqlConnection(conString);
try
{
con.Open();
SqlCommand com = new SqlCommand(sql, con);
com.Parameters.AddRange(sp);
return com.ExecuteReader(CommandBehavior.CloseConnection);
}
catch (Exception ex)
{
con.Close();
throw ex;
}
}
//返回数据集
public DataSet ExecuteAdater(string sql, params SqlParameter[] sp)
{
SqlConnection con = new SqlConnection(conString);
try
{
SqlCommand com = new SqlCommand(sql, con);
com.Parameters.AddRange(sp);
SqlDataAdapter adapter = new SqlDataAdapter(com);
DataSet ds = new DataSet();
adapter.Fill(ds, "a");
return ds;
}
catch (Exception)
{
throw;
}
finally
{
con.Close();
}
}
//返回受影响行数 (处理增删改)
public int ExecuteNonQuery(string sql, CommandType type = CommandType.Text, params SqlParameter[] sp)
{
SqlConnection con = new SqlConnection(conString);
try
{
con.Open();
SqlCommand com = new SqlCommand(sql, con);
com.Parameters.AddRange(sp);
com.CommandType = type;
Console.WriteLine(com);
return com.ExecuteNonQuery();
}
catch (Exception)
{
con.Close();
throw;
}
finally
{
con.Close();
}
}
}
版权声明:本文为saga661原创文章,遵循 CC 4.0 BY-SA 版权协议,转载请附上原文出处链接和本声明。