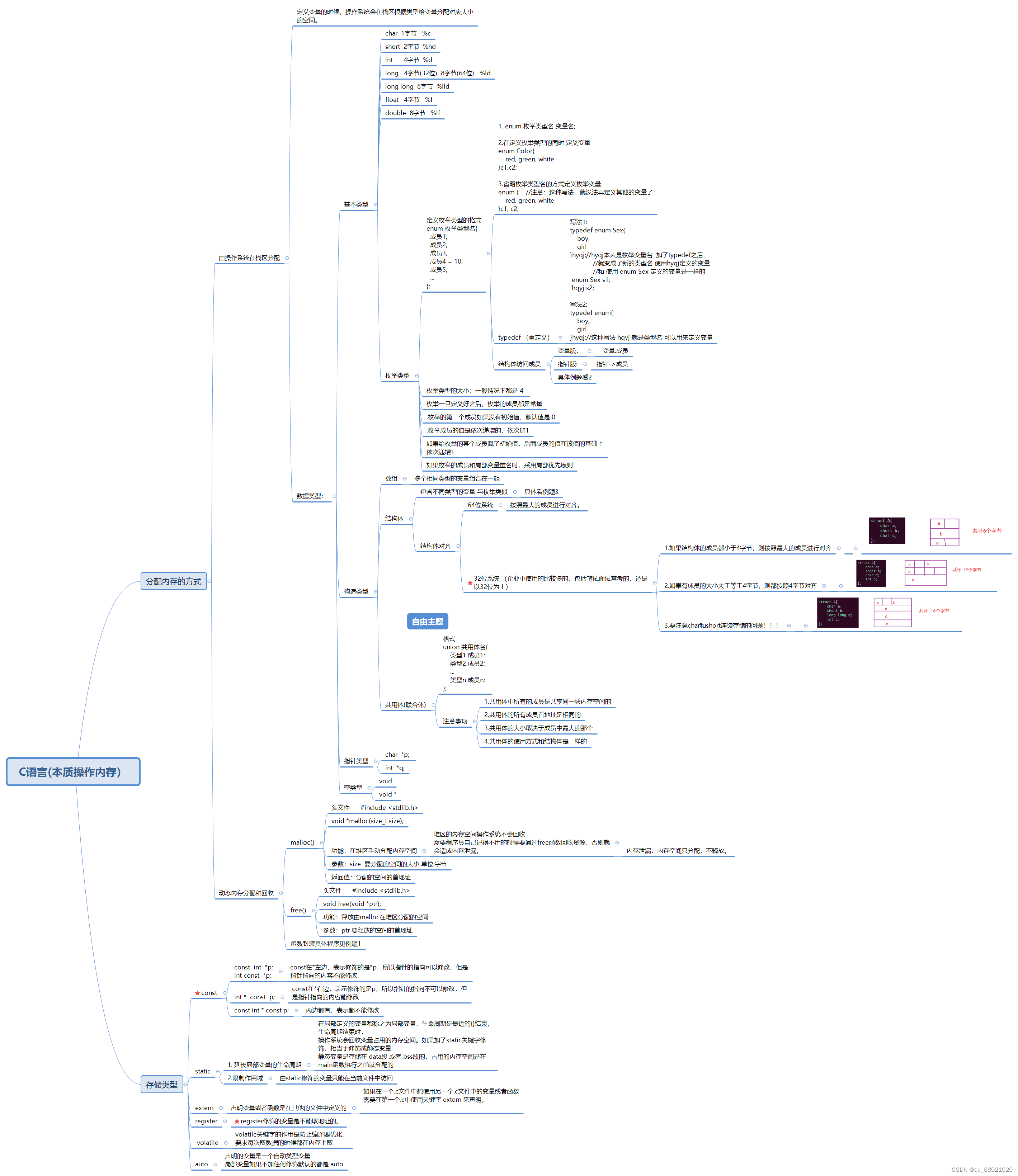
1.
自己封装一个函数 my_malloc,函数的功能是能在堆区分配 5个元素的int数组 大小的空间
再封装一个函数 my_free,能释放分配的空间
给数组的5个元素都赋值 在遍历输出一次。调用并测试。
#include <stdio.h>
#include <stdlib.h>
void my_malloc1(int **p){
*p = (int *)malloc(sizeof(int)*5);
printf("p = %p\n", *p);
}
void my_free2(int **p){
free(*p);
*p = NULL;
}
int main(){
int *s = NULL;
my_malloc1(&s);//地址传参
printf("s = %p\n", s);
s[0] = 520;
*(s+1) = 1314;
printf("%d\n", s[0]);//520
printf("%d\n", s[1]);//1314
my_free2(&s);
printf("s = %p\n", s);
return 0;
}
2. 枚举的基本使用
#include <stdio.h>
enum Color{
red,
white,
black
}c1;
enum{
boy,
girl
}sex;
int main(int argc, const char *argv[])
{
c1 = red;
printf("c1 = %d\n", red);//0
enum Color c2 = white;
printf("c2 = %d\n", c2);//1
sex = boy;
printf("sex = %d\n", sex);//0
return 0;
}
3.struct封装函数指针的使用
#include <stdio.h>
#include <stdlib.h>
struct Test{
int value;
int (*func)(int, int);//指针函数
};
int my_add(int x, int y){
return x+y;
}
int my_sub(int x, int y){
return x-y;
}
int main(int argc, const char *argv[])
{
struct Test t1;
t1.func = my_add;
struct Test *t2 = (struct Test *)malloc(sizeof(struct Test));
t2->func = my_sub;
printf("%d\n", t1.func(10, 20));//30
printf("%d\n", t2->func(10, 20));//-10
return 0;
}
版权声明:本文为qq_68021020原创文章,遵循 CC 4.0 BY-SA 版权协议,转载请附上原文出处链接和本声明。