一、项目介绍
简单介绍一下我写这个项目的思路,这里我使用的是MVC设计模式
M:Model(模型层)也叫数据层,在这里进行数据的操作。
V:Views(视图层),在这里进行界面以及提示信息的显示。
C:Controller(控制器),这里进行流程调度
项目比较入门适合刚学习JAVA的小伙伴进行查看
二、项目功能
在这里只做了增、删、改、查四种操作
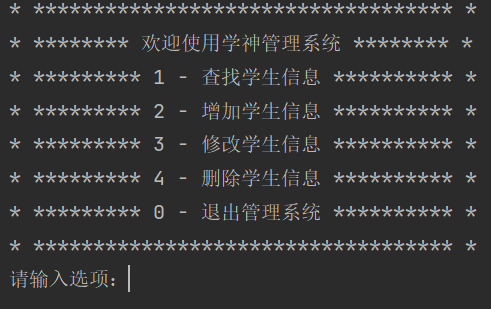
1、查找学生信息(效果图)
这里显示的是查找功能的二级菜单
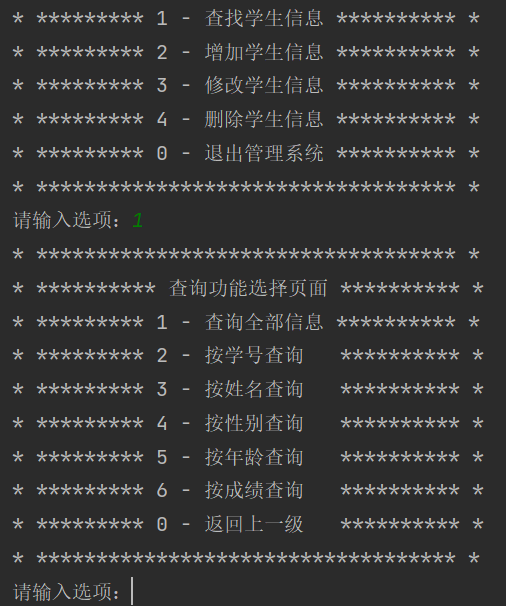
这里按学号查询
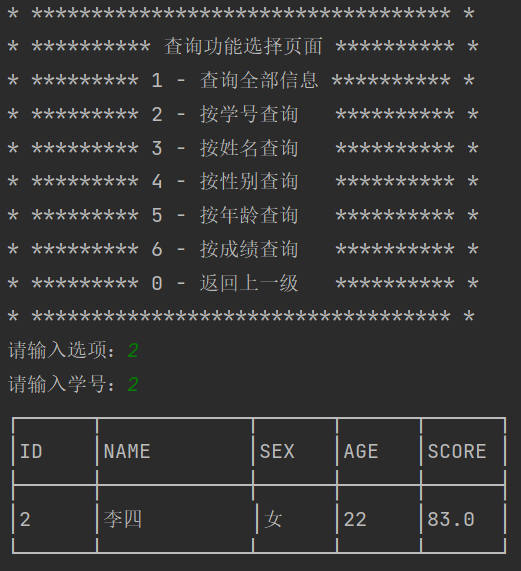
2、添加学生信息(效果图)
这里展示一下添加学生信息
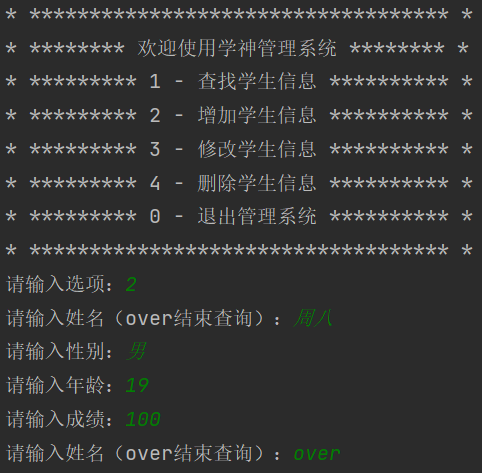
查找之后显示添加成功
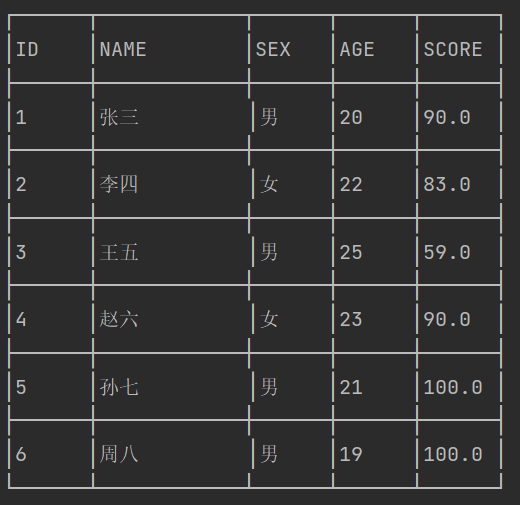
3、修改学生信息(效果图)
展示一下修改学生信息
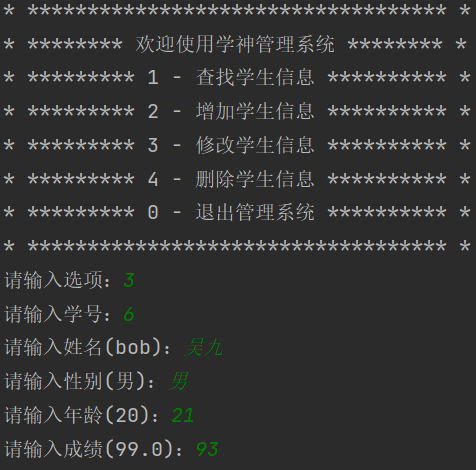
查找之后显示成功
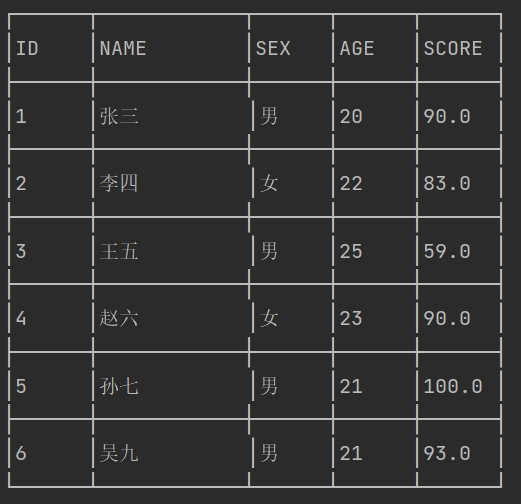
4、删除学生信息(效果图)
展示一下删除学生信息
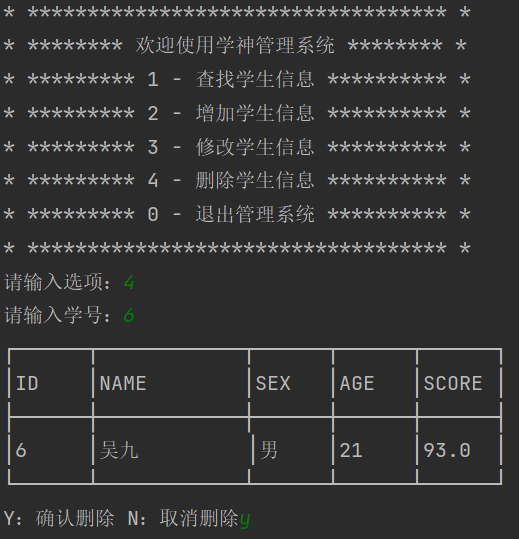
查找之后显示成功
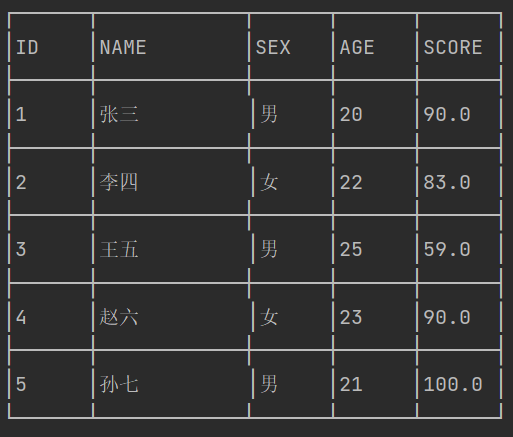
三、项目设计流程(附原码)
这是项目所用到的全部类
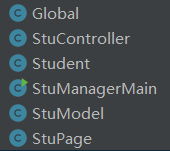
Global:因为比较入门没有用到数据库,只是简单的用集合来存储用户数据
StuController:这个里面是一些项目功能的调度器
Student:学生类
StuManagerMain:启动项
StuModel:用户模型层,用来处理用户数据
StuPage:视图层,用来显示界面、用户信息、提示信息
设计思路:首先通过视图层显示用户界面并获取用户选择的操作数,将操作数传入调度器中,根据操作数通过调度器来进行程序调度,调度器调用模型层进行数据操作,将操作好的数据返回调度器并通过视图层将数据显示出来,这就是基本的MVC设计模式。
1、Student.java
package com.kxs.demo02;
/**
* @ClassName Student
* @Description: TODO 学生类
* @Author: 2119475835@qq.com
* @Date: 2022/11/6
*/
public class Student {
private int id;
private String name;
private String sex;
private int age;
private float score;
public Student() {
}
public Student(int id, String name, String sex, int age, float score) {
this.id = id;
this.name = name;
this.sex = sex;
this.age = age;
this.score = score;
}
public Student(String name, String sex, int age, float score) {
this.name = name;
this.sex = sex;
this.age = age;
this.score = score;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public float getScore() {
return score;
}
public void setScore(float score) {
this.score = score;
}
@Override
public String toString() {
return "Student{" +
"id=" + id +
", name='" + name + '\'' +
", sex='" + sex + '\'' +
", age=" + age +
", score=" + score +
'}';
}
}
2、StuManagerMain.java
package com.kxs.demo02;
import java.io.IOException;
/**
* @ClassName StuManagerMain
* @Description: TODO 主类 测试类
* @Author: 2119475835@qq.com
* @Date: 2022/11/6
*/
public class StuManagerMain {
// 实例化一个控制器对象
private static StuController sc = new StuController();
public static void main(String[] args) throws IOException {
// 调用 Global 类当中的测试数据初始化方法来初始化学生信息数据集合
Global.initStuList();
// 输出欢迎页面,并让用户选择要操作的功能,获取到对应的序号
while(true){
int a = StuPage.welcome();
sc.action(a);
} // 在一个死循环里做程序的操作功能
}
}
3、StuPage.java
package com.kxs.demo02;
import java.util.ArrayList;
import java.util.Scanner;
/**
* @ClassName StuPage
* @Description: TODO 页面类 视图层 数据的展示与请求数据的获取
* @Author: 2119475835@qq.com
* @Date: 2022/11/6
*/
public class StuPage {
// 构造方法私有化,禁止在类外进行实例化对象
private StuPage(){}
public static int welcome() {
System.out.println("* *********************************** *");
System.out.println("* ******** 欢迎使用学神管理系统 ******** *");
System.out.println("* ********* 1 - 查找学生信息 ********** *");
System.out.println("* ********* 2 - 增加学生信息 ********** *");
System.out.println("* ********* 3 - 修改学生信息 ********** *");
System.out.println("* ********* 4 - 删除学生信息 ********** *");
System.out.println("* ********* 0 - 退出管理系统 ********** *");
System.out.println("* *********************************** *");
Scanner sc = new Scanner(System.in);
int a;
do {
System.out.print("请输入选项:");
a = sc.nextInt();
if(a < 5 && a >= 0){
return a;
}else{
System.out.println("请输入正确的选项,请重新输入");
}
}while(true);
}
/**
* 查询的二级菜单页面
* @return 用户请求
*/
public static int selShowBy() {
System.out.println("* *********************************** *");
System.out.println("* ********** 查询功能选择页面 ********** *");
System.out.println("* ********* 1 - 查询全部信息 ********** *");
System.out.println("* ********* 2 - 按学号查询 ********** *");
System.out.println("* ********* 3 - 按姓名查询 ********** *");
System.out.println("* ********* 4 - 按性别查询 ********** *");
System.out.println("* ********* 5 - 按年龄查询 ********** *");
System.out.println("* ********* 6 - 按成绩查询 ********** *");
System.out.println("* ********* 0 - 返回上一级 ********** *");
System.out.println("* *********************************** *");
Scanner sc = new Scanner(System.in);
int a;
do {
System.out.print("请输入选项:");
a = sc.nextInt();
if(a < 7 && a >= 0){
return a;
}else{
System.out.println("请输入正确的选项,请重新输入");
}
}while(true);
}
/**
* 显示学生信息
* @param stuList 学员信息集合
*/
public static void showStuList(ArrayList<Student> stuList) {
System.out.printf("┌──────┬────────────┬──────┬──────┬──────┐\n");
System.out.printf("│%-6s│%-12s│%-6s│%-6s│%-6s│\n", "ID", "NAME", "SEX", "AGE", "SCORE");
for (Student stu : stuList){
System.out.printf("├──────┼────────────┼──────┼──────┼──────┤\n");
System.out.printf("│%-6d│%-11s│%-5s│%-6d│%-6.1f│\n", stu.getId(),
stu.getName(),
stu.getSex(),
stu.getAge(),
stu.getScore());
}
System.out.printf("└──────┴────────────┴──────┴──────┴──────┘\n");
if(stuList.size() == 0){
System.out.println("对不起,没有找到该同学!");
}
}
/**
* 获取要查询的学生ID
* @return 学员ID
*/
public static int getStuID() {
System.out.print("请输入学号:");
return new Scanner(System.in).nextInt();
}
/**
* 获取要查询的学生姓名
* @return 学生姓名
*/
public static String getStuName() {
System.out.print("请输入要查找的姓名:");
return new Scanner(System.in).nextLine();
}
/**
* 获取要查询的学生性别
* @return 学生性别
*/
public static String getStuSex() {
Scanner sc = new Scanner(System.in);
do {
System.out.print("请输入要查找的性别:");
String stuSex = sc.nextLine();
if(stuSex.equals("男") || stuSex.equals("女")){
return stuSex;
}
}while(true);
}
/**
* 获取要查询的学生年龄
* @return 学生年龄
*/
public static int getStuAge() {
Scanner sc = new Scanner(System.in);
do {
System.out.print("请输入要查找的年龄:");
int stuAge = sc.nextInt();
if(stuAge < 100 && stuAge > 0){
return stuAge;
}
}while(true);
}
/**
* 获取要查询的学生成绩
* @return 学生成绩
*/
public static float getStuScore() {
Scanner sc = new Scanner(System.in);
do {
System.out.print("请输入要查找的成绩:");
float stuScore = sc.nextFloat();
if(stuScore <= 100 && stuScore >= 0){
return stuScore;
}
}while(true);
}
/**
* 获取一个学员对象,用于添加到学生集合里
* @return 用户输入学员信息 - 不包含I
*/
public static Student getAddStu() {
System.out.print("请输入姓名(over结束查询):");
String name = new Scanner(System.in).nextLine();
if (name.equals("over")){
return null;
}
System.out.print("请输入性别:");
String sex = new Scanner(System.in).nextLine();
System.out.print("请输入年龄:");
int age = new Scanner(System.in).nextInt();
System.out.print("请输入成绩:");
float score = new Scanner(System.in).nextFloat();
return new Student(name, sex, age, score);
}
public static boolean delAlert() {
System.out.print("Y:确认删除 N:取消删除");
switch(new Scanner(System.in).next()){
case "Y":
case "y":
return true;
case "N":
case "n":
return false;
default:
System.out.println("输入非法,取消删除");
return false;
}
}
public static void failed(String str) {
System.out.println("删除失败" + str);
}
public static Student editStu(Student stu) {
System.out.print("请输入姓名("+stu.getName()+"):");
String name = new Scanner(System.in).nextLine();
System.out.print("请输入性别("+stu.getSex()+"):");
String sex = new Scanner(System.in).nextLine();
System.out.print("请输入年龄("+stu.getAge()+"):");
int age = new Scanner(System.in).nextInt();
System.out.print("请输入成绩("+stu.getScore()+"):");
float score = new Scanner(System.in).nextFloat();
return new Student(stu.getId(), name, sex, age, score);
}
}
4、StuController.java
package com.kxs.demo02;
import java.util.ArrayList;
/**
* @ClassName StuController
* @Description: TODO 功能调度 控制器
* @Author: 2119475835@qq.com
* @Date: 2022/11/6
*/
public class StuController {
private StuModel sm = new StuModel();
/**
* 总控制器,功能调度方法
*
* @param a 用户的请求数据 - 用户选择的功能
*/
public void action(int a) {
switch (a) {
case 1:
// 定义一个二级页面
// 通过二级页面获取一个查询请求数据
int sa = StuPage.selShowBy();
// 查询功能调度
selAction(sa);
break;
case 2:
// (添加一个,添加多个)
// 获取要添加的学生对象
// Student stuAdd = StuPage.getAddStu();
// 将对象传递给 Model 层来实现添加
while(sm.doAddStu(StuPage.getAddStu()));
break;
case 3:
// 查找要修改的学员ID
int editStuID = StuPage.getStuID();
// 通过ID查询出要修改的学员信息
ArrayList<Student> editStu = sm.getStuByID(editStuID);
// 通过编辑页面将修改后的信息存放到新的对象中
Student stuNew = StuPage.editStu(editStu.get(0));
// 更新信息
sm.doUpdateStu(stuNew);
break;
case 4:
// 获取要删除的ID
int delID = StuPage.getStuID();
ArrayList<Student> delStu = sm.getStuByID(delID);
// 确认是否删除该信息Y:确认 / N:取消
if(!delStu.isEmpty()){
StuPage.showStuList(delStu);
// true 表示删除,false 表示取消
if(StuPage.delAlert()){
sm.doDelStuByID(delID);
}
}else{
StuPage.failed("要删除的学员信息不存在");
}
break;
case 0:
System.out.println("退出");
System.exit(0);
break;
}
}
/**
* 查询学生信息的二级菜单控制器
* @param sa 用户的操作数据
*/
private void selAction(int sa) {
switch (sa) {
case 1: // 查询全部
// 获取所有学生的信息
ArrayList<Student> stuList = sm.getAll();
// 通过页面输出
StuPage.showStuList(stuList);
break;
case 2: // 学号
// 获取学号
int StuID = StuPage.getStuID();
// 通过学号获取学生信息
ArrayList<Student> stuListById= sm.getStuByID(StuID);
// 显示学生信息
StuPage.showStuList(stuListById);
break;
case 3: // 姓名
// 获取姓名
String stuName= StuPage.getStuName();
// 通过姓名获取学生信息
ArrayList<Student> stuListByName = sm.getStuByName(stuName);
// 显示学生信息
StuPage.showStuList(stuListByName);
break;
case 4: // 性别
// 获取性别
String stuSex = StuPage.getStuSex();
// 通过性别获取学生信息
ArrayList<Student> stuListBySex = sm.getStuBySex(stuSex);
// 显示学生信息
StuPage.showStuList(stuListBySex);
break;
case 5: // 年龄
// 获取年龄
int stuAge = StuPage.getStuAge();
// 根据年龄获取学生信息
ArrayList<Student> stuListByAge = sm.getStuByAge(stuAge);
// 显示学生信息
StuPage.showStuList(stuListByAge);
break;
case 6: // 成绩
// 获取成绩
float stuScore = StuPage.getStuScore();
// 根据成绩获取学生信息
ArrayList<Student> stuListByScore = sm.getStuByScore(stuScore);
// 显示学生信息
StuPage.showStuList(stuListByScore);
break;
case 0: // 返回上一级
int a = StuPage.welcome();
action(a);
break;
}
}
}
5、StuModel.java
package com.kxs.demo02;
import java.util.ArrayList;
/**
* @ClassName StuModel
* @Description: TODO 数据处理 模型层
* @Author: 2119475835@qq.com
* @Date: 2022/11/6
*/
public class StuModel {
/**
* 获取全部学生信息
* @return 全部学生信息的对象
*/
public ArrayList<Student> getAll() {
return Global.stuList;
}
/**
* 根据ID获取对应学生信息
* @param stuID 学生ID
* @return 学生信息集合
*/
public ArrayList<Student> getStuByID(int stuID) {
ArrayList<Student> resList = new ArrayList<>();
for (Student stu : Global.stuList){
if(stuID == stu.getId()){
resList.add(stu);
}
}
return resList;
}
/**
* 根据姓名获取对应学生信息
* @param stuName 学生姓名
* @return 学生信息集合
*/
public ArrayList<Student> getStuByName(String stuName) {
ArrayList<Student> resList = new ArrayList<>();
for(Student stu : Global.stuList){
if(stuName.equals(stu.getName())){
resList.add(stu);
}
}
return resList;
}
/**
* 根据性别获取对应学生信息
* @param stuSex 学生性别
* @return 学生信息集合
*/
public ArrayList<Student> getStuBySex(String stuSex) {
ArrayList<Student> resList = new ArrayList<>();
for(Student stu : Global.stuList){
if(stuSex.equals(stu.getSex())){
resList.add(stu);
}
}
return resList;
}
/**
* 根据年龄获取对应学生信息
* @param stuAge 学生年龄
* @return 学生信息集合
*/
public ArrayList<Student> getStuByAge(int stuAge) {
ArrayList<Student> resList = new ArrayList<>();
for(Student stu : Global.stuList){
if(stuAge == stu.getAge()){
resList.add(stu);
}
}
return resList;
}
/**
* 根据成绩获取对应学生信息
* @param stuScore 学生成绩
* @return 学生信息集合
*/
public ArrayList<Student> getStuByScore(float stuScore) {
ArrayList<Student> resList = new ArrayList<>();
for(Student stu : Global.stuList){
if(stuScore == stu.getScore()){
resList.add(stu);
}
}
return resList;
}
/**
* 添加学员信息
* @param stuAdd 要被添加的学员信息,不包含ID的信息
* @return 成功返回true,失败返回false
*/
public boolean doAddStu(Student stuAdd) {
if(stuAdd == null){
return false;
}
Student stu = new Student(Global.stuId++,
stuAdd.getName(),
stuAdd.getSex(),
stuAdd.getAge(),
stuAdd.getScore());
return Global.stuList.add(stu);
}
/**
* 根据学生ID删除学员
* @param delID 要删除的学员ID
*/
public void doDelStuByID(int delID) {
for (int i = 0; i < Global.stuList.size(); i++) {
if(delID == Global.stuList.get(i).getId()){
Global.stuList.remove(i);
}
}
}
/**
* 更新学员
* @param stuNew 要更新的学员信息
*/
public void doUpdateStu(Student stuNew) {
for (int i = 0; i < Global.stuList.size(); i++) {
if(stuNew.getId() == Global.stuList.get(i).getId()){
Global.stuList.set(i, stuNew);
return;
}
}
}
}
6、Global.java
package com.kxs.demo02;
import java.util.ArrayList;
/**
* @ClassName Global
* @Description: TODO 全局类,定义一些功能性的方法
* @Author: 2119475835@qq.com
* @Date: 2022/11/6
*/
public class Global {
public static int stuId = 1;
public static ArrayList<Student> stuList = new ArrayList<>();
// 构造方法私有化,禁止在类外对其进行实例化
private Global(){
}
public static void initStuList() {
stuList.add(new Student(stuId++, "张三", "男", 20, 90f));
stuList.add(new Student(stuId++, "李四", "女", 22, 83f));
stuList.add(new Student(stuId++, "王五", "男", 25, 59f));
stuList.add(new Student(stuId++, "赵六", "女", 23, 90f));
stuList.add(new Student(stuId++, "孙七", "男", 21, 100f));
}
}
四、总结
这个项目适合刚学习JAVA的小伙伴做一做,弄明白了这个设计思路后可以试着根据一些自己的想法来试着做一下,欢迎大家指出问题,互相交流。
tips:
史上最详细的JAVA学生信息管理系统(MySQL实现)
这个是我写的升级版项目,欢迎大家阅读,互相学习!!!