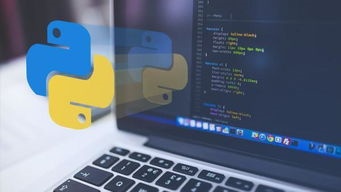
1、find
功能:用于在一段程序语句中,查找单个语句构成元素的位置或索引。
例如:
test = "abcd"
print(test.find('ab'))
输出结果为:0
2、split
功能:对一段程序语句进行分割处理,转换为列表的格式。
例如:
test = "a,b,c,d"
print(test.split(','))
输出结果为:['a', 'b', 'c', 'd']
3、replsce
功能:对一段程序语句中的构成元素进行替换。
例如:
string = "Python is good"
print(string.replace('Python','java'))
输出结果为:java is good
4、startswith
功能:用于检验在一段程序语句中,是否以特定元素(元素可以是单词、字母或数字)开头。
例如:
string = "this is a book"
print(string.startswith('this'))
输出结果为:True
5、endswith
功能:用于检验在一段程序语句中,是否以特定元素(可以是单词、字母或数字)结尾。
例如:
string = "this is a book"
print(string.endswith('book'))
输出结果为:True
6、strip()
功能:用于删除一段程序语句末尾或开头的换行符。
例如:
string = "this is a bookn"
print(string.strip())
输出结果为:this is a book
7、[::-1]
功能:用于倒转列表元素。
例如:
list = [1,2,3,4,5]
print(list[::-1])
输出结果为:[5, 4, 3, 2, 1]
8、sort(reverse = True)
功能:倒转列表元素。(一般与’ sort() ‘函数集合在一起使用)
例如:
list = [1,2,3,4,5]
print(list.sort(reverse = True))
输出结果为:[5, 4, 3, 2, 1]
9、rstrip()
功能:删除后空格。
例如:
>>> news = "I love you Python "
>>> news.rstrip()
'I love you Python'
备注:类似的还有“ lstrip (删除前空格) ”、“ strip (删除前后空格) ” 用法和格式与strip()一样.
10、import math math.sqrt()
功能:计算一个带根号的数值。
例如:
# 计算根号16的值.
import math
print(math.sqrt(16))
输出结果为:4.0